主题
Qwen-Agent
项目地址:https://github.com/QwenLM/Qwen-Agent
Qwen-Agent 是一个基于 Qwen 的指令遵循、工具使用、规划和记忆能力的 LLM 应用程序开发框架。
它还附带了一些示例应用程序,如浏览器助手、代码解释器和自定义助手。现在 Qwen-Agent 作为 Qwen Chat 的后端。
以下示例基于官方修改
python
import pprint
import urllib.parse
import json5
from qwen_agent.agents import Assistant
from qwen_agent.tools.base import BaseTool, register_tool
from qwen_agent.utils.output_beautify import typewriter_print
# Step 1 (Optional): Add a custom tool named `my_image_gen`.
@register_tool('my_image_gen')
class MyImageGen(BaseTool):
# The `description` tells the agent the functionality of this tool.
description = 'AI painting (image generation) service, input text description, and return the image URL drawn based on text information.'
# The `parameters` tell the agent what input parameters the tool has.
parameters = [{
'name': 'prompt',
'type': 'string',
'description': 'Detailed description of the desired image content, in English',
'required': True
}]
def call(self, params: str, **kwargs) -> str:
# `params` are the arguments generated by the LLM agent.
prompt = json5.loads(params)['prompt']
prompt = urllib.parse.quote(prompt)
return json5.dumps(
{'image_url': f'https://image.pollinations.ai/prompt/{prompt}'},
ensure_ascii=False)
# Step 2: Configure the LLM you are using.
llm_cfg = {
# Use the model service provided by DashScope:
'model': 'qwen-max-latest',
'model_server': 'dashscope',
# 'api_key': 'YOUR_DASHSCOPE_API_KEY',
# It will use the `DASHSCOPE_API_KEY' environment variable if 'api_key' is not set here.
# Use a model service compatible with the OpenAI API, such as vLLM or Ollama:
# 'model': 'Qwen2.5-7B-Instruct',
# 'model_server': 'http://localhost:8000/v1', # base_url, also known as api_base
# 'api_key': 'EMPTY',
# (Optional) LLM hyperparameters for generation:
'generate_cfg': {
'top_p': 0.8
}
}
# Step 3: Create an agent. Here we use the `Assistant` agent as an example, which is capable of using tools and reading files.
system_instruction = '''You are a helpful assistant.
After receiving the user's request, you should:
- first draw an image and obtain the image url,
- then run code `request.get(image_url)` to download the image,
- and finally select an image operation from the given document to process the image.
Please show the image using `plt.show()`.'''
tools = ['my_image_gen', 'code_interpreter'] # `code_interpreter` is a built-in tool for executing code.
files = ['doc.pdf'] # Give the bot a PDF file to read.
bot = Assistant(llm=llm_cfg,
system_message=system_instruction,
function_list=tools,
files=files)
# Step 4: Run the agent as a chatbot.
messages = [] # This stores the chat history.
while True:
# For example, enter the query "draw a dog and rotate it 90 degrees".
query = input('\nuser query: ')
# Append the user query to the chat history.
messages.append({'role': 'user', 'content': query})
response = []
response_plain_text = ''
print('bot response:')
for response in bot.run(messages=messages):
# Streaming output.
response_plain_text = typewriter_print(response, response_plain_text)
# Append the bot responses to the chat history.
messages.extend(response)
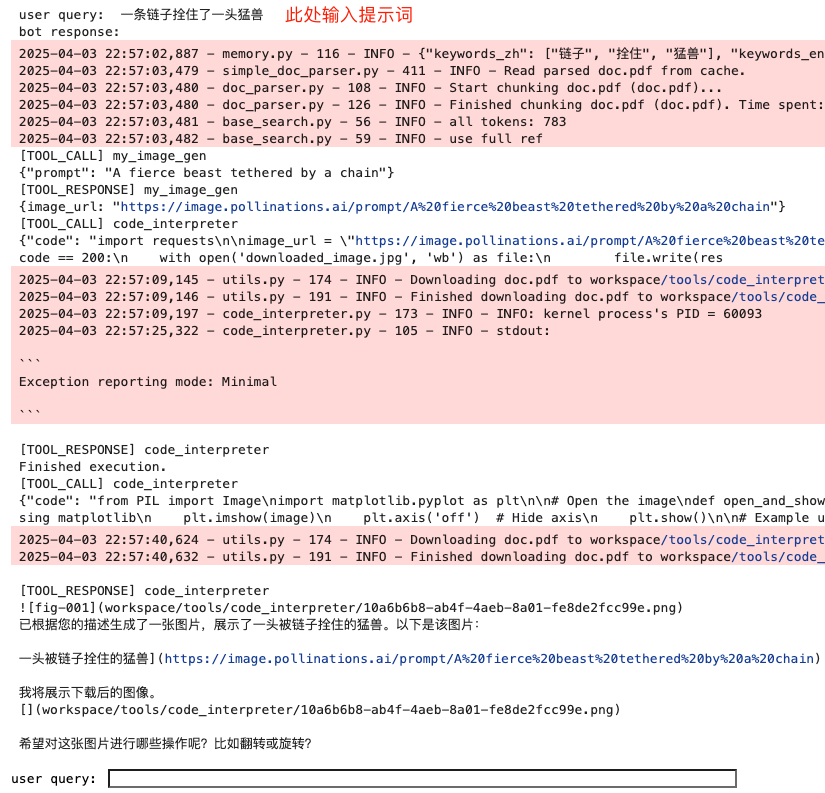
执行结果
user query: 一条链子拴住了一头猛兽
bot response:
2025-04-03 22:57:02,887 - memory.py - 116 - INFO - {"keywords_zh": ["链子", "拴住", "猛兽"], "keywords_en": ["chain", "tie up", "fierce beast", "animal"], "text": "一条链子拴住了一头猛兽"}
2025-04-03 22:57:03,479 - simple_doc_parser.py - 411 - INFO - Read parsed doc.pdf from cache.
2025-04-03 22:57:03,480 - doc_parser.py - 108 - INFO - Start chunking doc.pdf (doc.pdf)...
2025-04-03 22:57:03,480 - doc_parser.py - 126 - INFO - Finished chunking doc.pdf (doc.pdf). Time spent: 0.00013208389282226562 seconds.
2025-04-03 22:57:03,481 - base_search.py - 56 - INFO - all tokens: 783
2025-04-03 22:57:03,482 - base_search.py - 59 - INFO - use full ref
[TOOL_CALL] my_image_gen
{"prompt": "A fierce beast tethered by a chain"}
[TOOL_RESPONSE] my_image_gen
{image_url: "https://image.pollinations.ai/prompt/A%20fierce%20beast%20tethered%20by%20a%20chain"}
[TOOL_CALL] code_interpreter
{"code": "import requests\n\nimage_url = \"https://image.pollinations.ai/prompt/A%20fierce%20beast%20tethered%20by%20a%20chain\"\nresponse = requests.get(image_url)\n\nif response.status_code == 200:\n with open('downloaded_image.jpg', 'wb') as file:\n file.write(res
2025-04-03 22:57:09,145 - utils.py - 174 - INFO - Downloading doc.pdf to workspace/tools/code_interpreter/doc.pdf...
2025-04-03 22:57:09,146 - utils.py - 191 - INFO - Finished downloading doc.pdf to workspace/tools/code_interpreter/doc.pdf. Time spent: 0.000888824462890625 seconds.
2025-04-03 22:57:09,197 - code_interpreter.py - 173 - INFO - INFO: kernel process's PID = 60093
2025-04-03 22:57:25,322 - code_interpreter.py - 105 - INFO - stdout:
```
Exception reporting mode: Minimal
```
[TOOL_RESPONSE] code_interpreter
Finished execution.
[TOOL_CALL] code_interpreter
{"code": "from PIL import Image\nimport matplotlib.pyplot as plt\n\n# Open the image\ndef open_and_show_image(filename):\n image = Image.open(filename)\n \n # Display the image using matplotlib\n plt.imshow(image)\n plt.axis('off') # Hide axis\n plt.show()\n\n# Example usage\nopen_and_show_image('downloaded_image.
2025-04-03 22:57:40,624 - utils.py - 174 - INFO - Downloading doc.pdf to workspace/tools/code_interpreter/doc.pdf...
2025-04-03 22:57:40,632 - utils.py - 191 - INFO - Finished downloading doc.pdf to workspace/tools/code_interpreter/doc.pdf. Time spent: 0.005845069885253906 seconds.
[TOOL_RESPONSE] code_interpreter

已根据您的描述生成了一张图片,展示了一头被链子拴住的猛兽。以下是该图片:
一头被链子拴住的猛兽](https://image.pollinations.ai/prompt/A%20fierce%20beast%20tethered%20by%20a%20chain)
我将展示下载后的图像。
[](workspace/tools/code_interpreter/10a6b6b8-ab4f-4aeb-8a01-fe8de2fcc99e.png)
希望对这张图片进行哪些操作呢?比如翻转或旋转?
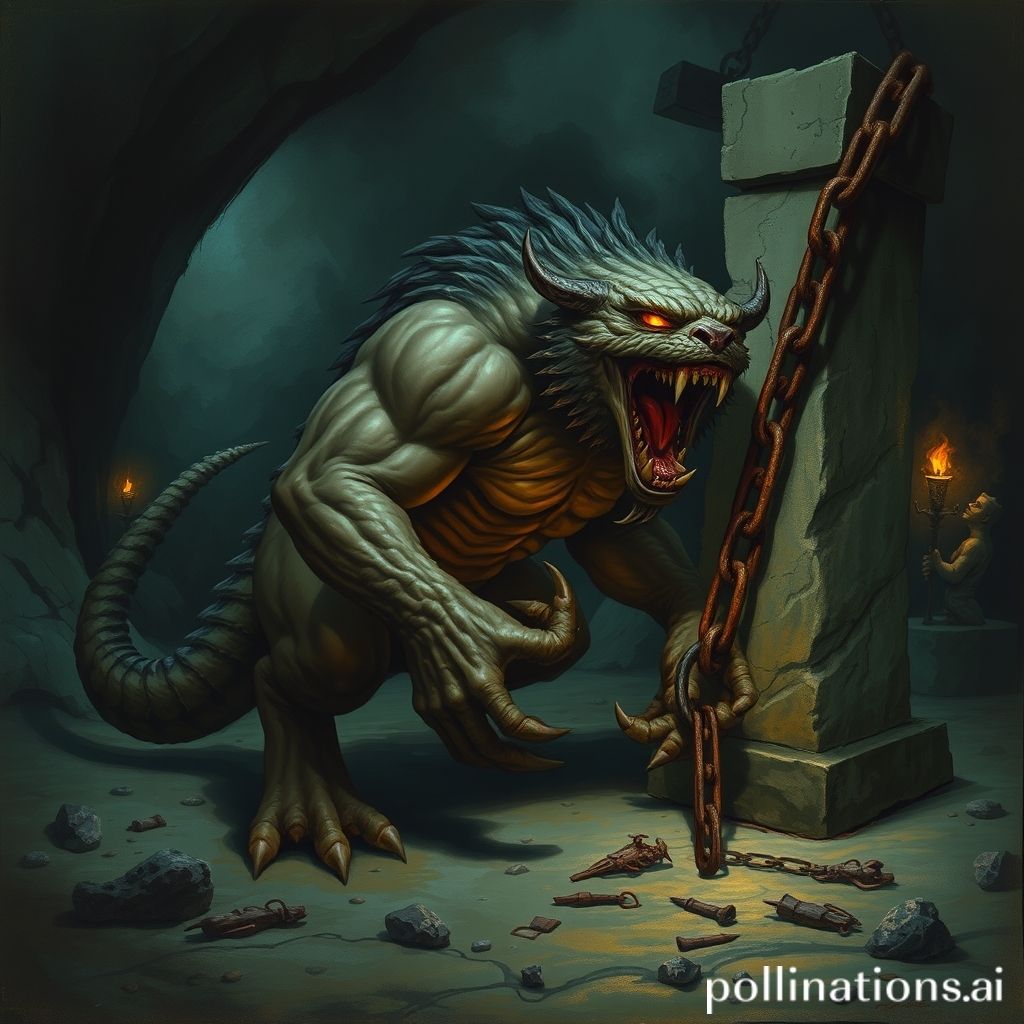
Agent执行的结果
shell
workspace
└── tools
├── code_interpreter
│ ├── 10a6b6b8-ab4f-4aeb-8a01-fe8de2fcc99e.png
│ ├── 4541f543-91d1-4050-98a3-7d83f62af592.png
│ ├── dd207beb-e097-452f-886c-79047772ddef.png
│ ├── doc.pdf
│ ├── downloaded_image.jpg
│ ├── kernel_connection_file_fc6f8461-90bc-4b35-a5fa-a910fed0b0b5_60065.json
│ └── launch_kernel_fc6f8461-90bc-4b35-a5fa-a910fed0b0b5_60065.py
├── doc_parser
│ └── 24cc42a6ca852ccdfaae715a9a9873dd03aa255ae8e213db8aeb720a8df2f947_without_chunking
└── simple_doc_parser
└── 24cc42a6ca852ccdfaae715a9a9873dd03aa255ae8e213db8aeb720a8df2f947_ori
WebUI
python
from qwen_agent.gui import WebUI
WebUI(bot).run() # bot is the agent defined in the above code, we do not repeat the definition here for saving space.
输出以下提示词
shell
日落余晖,多重树叶透光,胶片摄影,青橙色,情侣,隐约,光朦,高反差,高饱和,高逆光,高曝,多重曝光,氛围感拉满,层次感,梦幻,写意,奇妙意境,杰作,艺术英文信息解析点缀
安装方法
shell
git clone https://github.com/QwenLM/Qwen-Agent.git
cd Qwen-Agent
pip install -U "qwen-agent[gui,rag,code_interpreter,mcp]"